Blade Directives
Laravext offers some blade directives to be used in your project.
@nexus
The @nexus
directive is used to define where the content of the page.(jsx|tsx|js|ts|vue)
files (an all the outer file conventions) will be rendered. Assuming you have a ./resources/views/layouts/app.blade.php
blade view (with a @yield('content')
inside it), and a ./resources/views/sections/app.blade.php
you should use the @nexus
directive like this:
@extends('layouts.app')
@section('content')
@nexus
@endsection
@startNexus and @endNexus
As mentioned before in the Concepts/File Conventions/Loading section of this documentation, you might need more complex server side skeletons to be rendered while the javascript is being loaded (assuming you don't want to use the Javascript Runtime SSR), so you can use the @startNexus
and @endNexus
directives to define where the content of the page.(jsx|tsx|js|ts|vue)
files (an all the outer file conventions) will be rendered. Assuming you have a ./resources/views/layouts/app.blade.php
blade view (with a @yield('content')
inside it), and a ./resources/views/sections/app.blade.php
you should use the @startNexus
and @endNexus
directives like this:
@extends('layouts.app')
@section('content')
@startNexus
@auth
<h1 class="text-xl font-bold text-gray-800 dark:text-gray-200">Welcome back,
{{ auth()->user()->name }}</h1>
@endauth
@guest
<h1 class="text-xl font-bold text-gray-800 dark:text-gray-200">Welcome, stranger</h1>
@endguest
@endNexus
@endsection
Once again... Remember that anything in between those two directives will be cleared when the content of the page component is loaded.
@strand
You can use the @strand('Path/To/Component') directive alongside a @nexus, which will use the name as a path to find a React/Vue component inside the resources/js/strands (which is customizable at the createLaravextApp
/createLaravextSsrApp
functions). The first parameter is the path/to/the/component
, and the second one is ['any' => 'data', 'you' => 'might need']
@extends('layouts.app')
@section('content')
@strand('PrivacyToggle', ['initialState' => auth()->user()?->privacy ?? false])
@nexus
@endsection
While this package was being developed, it was noticed that if a @strand
directive was placed in a layout blade view, any parameter passed to it was cached,
so avoid using it in a layout blade view if you're passing any props to it. An example was that a date would always be "stuck" in the date when the project created cached view files.
For some context, here's the component:
- React
- Vue
- PrivacyToggle.jsx
- usePrivacy.js
import usePrivacy from '@/hooks/usePrivacy'
import axios from 'axios';
import { useEffect } from 'react'
export default ({ laravext, initialState }) => {
const { active, setActive, toggle } = usePrivacy();
useEffect(() => {
if(initialState !== undefined){
setActive(initialState);
}
}, []);
const handleToggle = () => {
// This is done like this because the active wouldn't always be updated immediately
let currentState = active;
toggle();
axios.put('/api/auth/user/privacy', { privacy: !currentState })
}
return (
<>
<span className="cursor-pointer" onClick={handleToggle}>{active ? 'Click to Turn Privacy Off' : 'Click to Turn Privacy On'}</span>
</>
)
}
⚠️This example uses the zustand package⚠️
import { create } from 'zustand'
const usePrivacy = create((set) => ({
active: false,
setActive: (active) => set(() => ({ active })),
toggle: () => set((state) => ({ active: !state.active })),
}))
export default usePrivacy;
- PrivacyToggle.vue
- usePrivacy.js
<script setup>
import { privacy } from '@/composables/usePrivacy'
import axios from 'axios';
const {initialState, laravext} = defineProps(['initialState', 'laravext'])
if(initialState !== undefined) {
privacy.setActive(initialState)
}
const handleToggle = () => {
privacy.toggle();
axios.put('/api/auth/user/privacy', {
privacy: privacy.active
});
}
</script>
<template>
<span @click="handleToggle" class="cursor-pointer">
{{ privacy.active ? 'Click to Turn Privacy Off' : 'Click to Turn Privacy On' }}
</span>
</template>
import { reactive } from 'vue'
export const privacy = reactive({
active: false,
toggle() {
this.active = !this.active
},
setActive(value) {
this.active = value
}
})
@startStrand and @endStrand
Similar to @strand
and @startNexus
and @endNexus
, you can use the @startStrand
and @endStrand
directives to create a section where it will render a React/Vue component inside the resources/js/strands (which is customizable at the createLaravextApp
/createLaravextSsrApp
functions). The first parameter is the path/to/the/component
, and the second one is ['any' => 'data', 'you' => 'might need']
, just like the @strand
directive. The difference is that you can use the @startStrand
and @endStrand
directives to insert a more complex server skeleton that will be displayed while the javascript is loading.
@extends('layouts.app')
@section('content')
@startStrand('NavBar', ['initialState' => auth()->user()?->privacy ?? false])
@auth
<nav class="text-xl font-bold text-gray-800 dark:text-gray-200">
<!-- Your navigation here -->
</nav>
@endauth
@guest
<nav class="text-xl font-bold text-gray-800 dark:text-gray-200">
<!-- Your navigation here -->
</nav>
@endguest
@endStrand
@endsection
Overview
In the end, depending on how/which directives you use, your overall blade structure might look like one of these illustrations:
@nexus
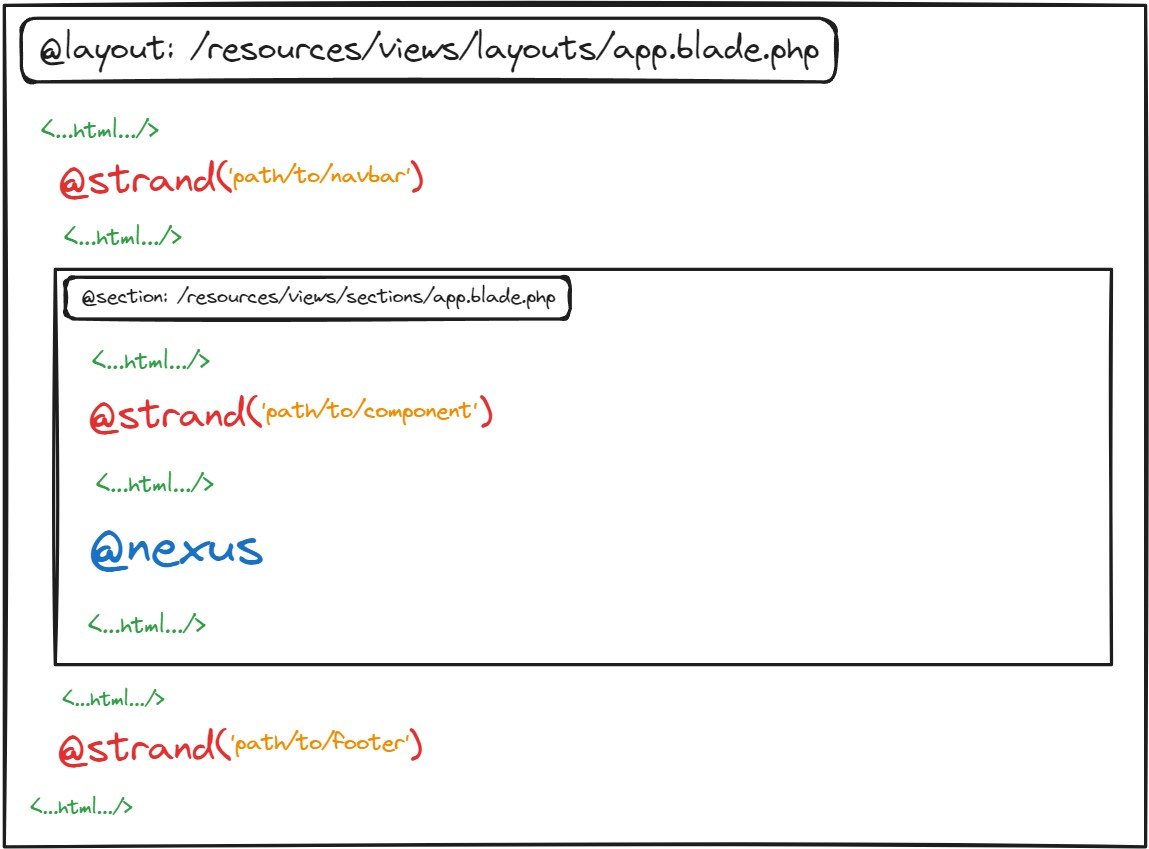
@startNexus and @endNexus
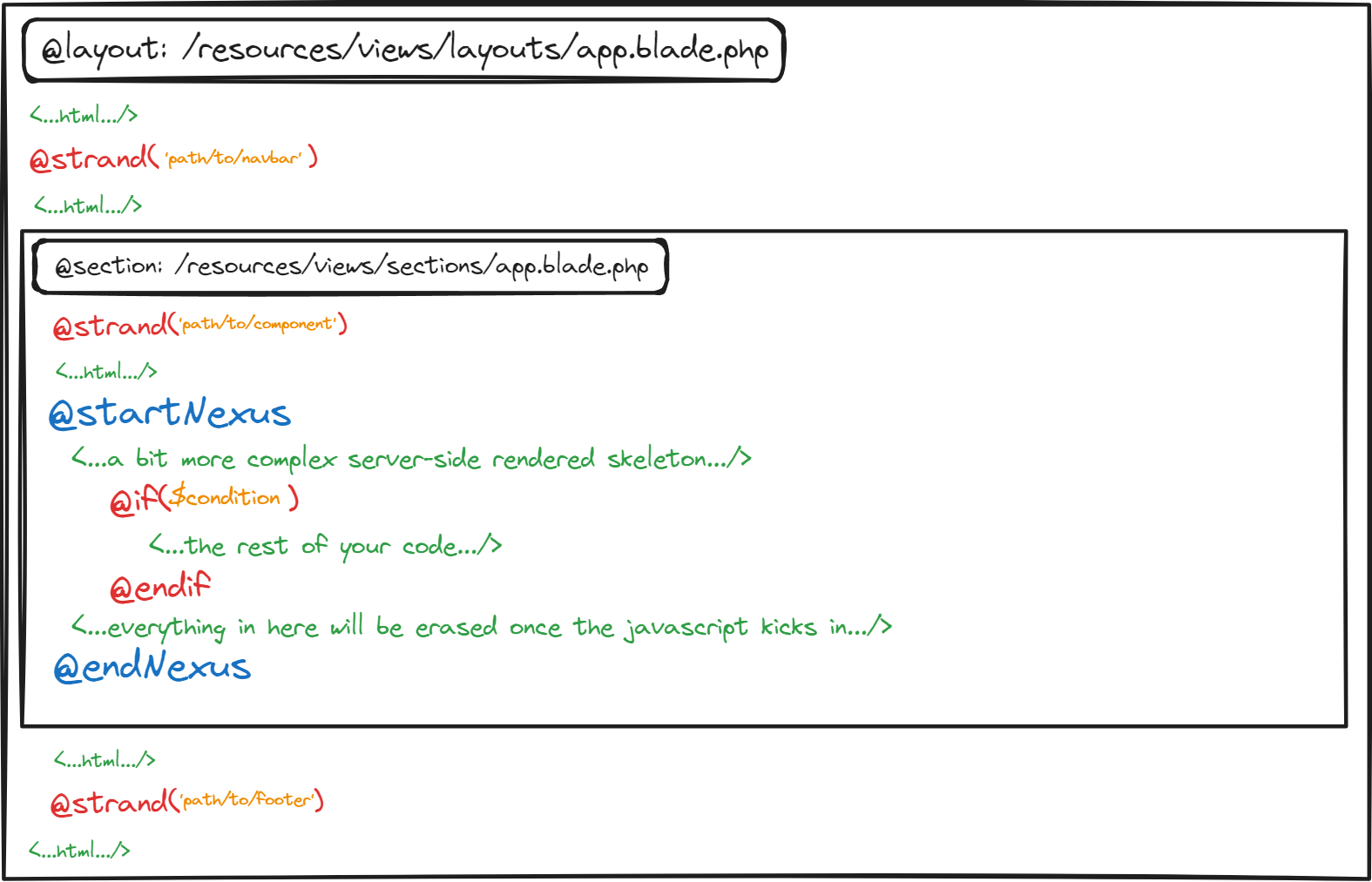
Just a reminder that the view file can be customized not only in the config file, but also in a more granular way, as shown in the Tools/Routing section.